Working Example
List to filter
Repeater: #repeater1
Replace an Inverter
Microinverter
Other
Replace an Inverter
Optimizer
Solar Edge
Replace an Inverter
Optimizer
Tigo
Replace a Solar Panel
Blue
Solar World
Replace a Solar Panel
Blue
TMI
Replace a Solar Panel
Black
Solar Edge
Replace an Inverter
Microinverter
Enphase
Replace an Inverter
String Inverter
SMA
Replace an Inverter
Microinverter
SMA
Replace an Inverter
String Inverter
Other
Replace an Inverter
String Inverter
Fromius
Replace a Solar Panel
Black
Test
Replace a Solar Panel
Orange
Workworld
Replace a Solar Panel
Orange
One Solar
Number of List items:
Dropdown: #serviceList
Button: #resetButton
Dropdown: #inverterTypeList
Dropdown: #supplierList
Text: #itemCount
14
Show Dataset ---->
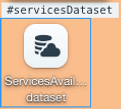

Page Code
// For full API documentation, including code examples, visit http://wix.to/94BuAAs
import wixData from 'wix-data';
// Javascript Class to manage dropdown data filtering
// When an object created from this class is given a dataset with the fields 'service', 'inverterType' and 'supplierName'
// The object will generate unique column lists formatted to be passed directly to a $w.DropDown.options property.
class dropDownData {
constructor(recordList = []) {
this.updateRecordList(recordList);
}
// Call this function to initialise the object data set and reset the column lists.
updateRecordList(recordList) {
this.list = recordList;
this.resetFieldLists();
}
resetFieldLists() {
this.services = [];
this.inverters = [];
this.suppliers = [];
}
// Returns a unique list based on the values in the services column
// The list contains objects with key value pairs for the keys 'label' and 'value'
servicesList() {
if (this.services.length === 0) {
// We haven't initialised serviceList yet yet so set it up
this.services = this.uniqueColumnListForField('service');
}
return this.services;
}
// Returns a unique list based on the values in the inverterType column
// The list contains objects with key value pairs for the keys 'label' and 'value'
invertersList() {
if (this.inverters.length === 0) {
// We haven't initialised inverterList yet yet so set it up
this.inverters = this.uniqueColumnListForField('inverterType');
}
return this.inverters;
}
// Returns a unique list based on the values in the supplierName column
// The list contains objects with key value pairs for the keys 'label' and 'value'
suppliersList() {
if (this.suppliers.length === 0) {
// We haven't initialised supplierList yet so set it up
this.suppliers = this.uniqueColumnListForField('supplierName');
}
return this.suppliers;
}
// Helper method for extracting column records and making them unique
// All list methods use this to generate list containing objects with
// key value pairs for the keys 'label' and 'value' which is expected
//by the $w.DropDown.options property
uniqueColumnListForField(field) {
let result = [];
let uniqueValues = []; // Used to ensure values in the list are unique
this.list.forEach(item => {
let fieldValue = item[field];
// Only store field values that we haven't yet seen. Skip null values
if (fieldValue && !uniqueValues.includes(fieldValue)) {
uniqueValues.push(fieldValue); // Remember this value to maintain uniqueness
// Save the resulting information in the format required by the DropDown options list.
result.push({'label':fieldValue, 'value':fieldValue});
}
});
​
// Return the list sorted by the label of the options list.
return sortObjectArrayBy(result, "label");
}
}
let dropDownRecords = new dropDownData();
$w.onReady(function () {
//We initialise the drop downs here once the data we need is available.
$w('#servicesDataset').onReady(() => {
updateDropDownOptions();
});
});
// We update the drop down lists from the currently filtered dataset.
// The data set is filtered by values from the drop down lists
function updateDropDownOptions() {
// Use getItems to load all items available from the filtered dataset
// When we apply a filter (see updateRepeaterFilter()) this gets called to reload the drop downs
$w('#servicesDataset').getItems(0,$w('#servicesDataset').getTotalCount())
.then((results) => {
// Update our dropdown object with the new data record list
dropDownRecords.updateRecordList(results.items);
// Update the counter on screen
$w('#itemCount').text = results.totalCount;
// Load/Reload dropdown data sets from our dropdown list data object
$w('#serviceList').options = dropDownRecords.servicesList();
$w('#inverterTypeList').options = dropDownRecords.invertersList();
$w('#supplierList').options = dropDownRecords.suppliersList();
});
}
// Function to filter dataset based upon the filter drop down values
function updateRepeaterFilter() {
// Empty filter will remove existing filters so if none of the dropdowns have values all
// Table records will be available to the repeater.
let repeaterFilter = wixData.filter();
// If we have any filter values we simply join them together using .eq()
if ($w('#serviceList').value) {
repeaterFilter = repeaterFilter.eq('service', $w('#serviceList').value);
}
if ($w('#inverterTypeList').value) {
repeaterFilter = repeaterFilter.eq('inverterType', $w('#inverterTypeList').value);
}
if ($w('#supplierList').value) {
repeaterFilter = repeaterFilter.eq('supplierName', $w('#supplierList').value);
}
// Update the filter on the dataset
$w('#servicesDataset').setFilter(repeaterFilter)
.then(() => {
// When we get here the dataset will be filtered so we need to reset the drop downs from the
// newly filtered record list
updateDropDownOptions();
});
}
// Helper functions triggered when we make a filter list selection in a drop down
export function serviceList_change(event) {
//Add your code for this event here:
updateRepeaterFilter();
}
export function inverterTypeList_change(event) {
//Add your code for this event here:
updateRepeaterFilter();
}
export function supplierList_change(event) {
//Add your code for this event here:
updateRepeaterFilter();
}
// Because the filter for the repeater uses multiple fields and these result in restricting the dropdown
// Option lists we need to reset the drop down lists to undefined to get all of the options back and
// repopulate the repeater.
// We could have a reset option as a button next to each drop down or add a drop down option of reset but this
// works for the purposes of this example
export function resetButton_click(event, $w) {
// Reset the filter list undefined forces the dropdown placeholder text to be displayed.
$w('#serviceList').selectedIndex = undefined;
$w('#inverterTypeList').selectedIndex = undefined;
$w('#supplierList').selectedIndex = undefined;
// With no drop down values selected the repeater filter will be cleared and all repeater items
// will be displayed
updateRepeaterFilter();
}
​
// Dedicated function that can be used to sort an array of objects. It takes the array and the name of the object property to sort by.
function sortObjectArrayBy(objectArray, objectProperty = "label") {
// Check if we have an array
let result = objectArray;
if (objectArray instanceof Array) {
// We have a valid Array lets get the list of properties
// We will use the sort function which uses a compare function to determine how to sort the array
result = objectArray.sort((a,b) => {
// a and b contain objects from the array to compare.
// We will sort by the objectProperty so extract the property value to compare with
let propertyA = a[objectProperty];
let propertyB = b[objectProperty];
let compareResult = 0; // Default the same
if (typeof propertyA === "string" && typeof propertyB === "string") {
// We have two values to compare
compareResult = propertyA.localeCompare(propertyB);
}
return compareResult;
});
}
return result;
}